UITableView 表格視圖
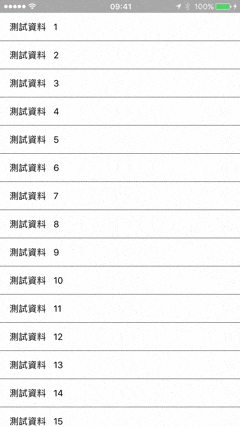
加入協定
class ViewController: UIViewController, UITableViewDelegate, UITableViewDataSource {
宣告資料
var tableView = UITableView()
var names = Array(1...20)
let WIDTH = UIScreen.main.bounds.size.width
let HEIGHT = UIScreen.main.bounds.size.height
viewDidLoad
view.backgroundColor = UIColor.lightGray
tableView = UITableView(frame: CGRect(x: 0,
y: 0,
width: WIDTH,
height: HEIGHT),
style: .plain)
tableView.delegate = self
tableView.dataSource = self
tableView.register(UITableViewCell.self,
forCellReuseIdentifier: "cell")
tableView.backgroundColor = UIColor.lightGray
tableView.separatorStyle = .singleLine
tableView.separatorColor = UIColor.lightGray
tableView.separatorInset = UIEdgeInsetsMake(0, 0, 0, 0)
tableView.scrollsToTop = false
tableView.tableFooterView = UIView(frame: .zero)
view.addSubview(tableView)
UITableViewDelegete、UITableViewDataSource
func numberOfSections(in tableView: UITableView) -> Int {
return 1
}
func tableView(_ tableView: UITableView, numberOfRowsInSection section: Int) -> Int {
return names.count
}
func tableView(_ tableView: UITableView, cellForRowAt indexPath: IndexPath) -> UITableViewCell {
var cell = tableView.dequeueReusableCell(withIdentifier: "cell")
if cell == nil {
cell = UITableViewCell(style: .default,
reuseIdentifier: "cell")
}
cell?.textLabel?.text = "測試資料 \(names[indexPath.row])"
return cell!
}
func tableView(_ tableView: UITableView, didSelectRowAt indexPath: IndexPath) {
tableView.deselectRow(at: indexPath,
animated: true)
}
更多常用設定
func tableView(_ tableView: UITableView, estimatedHeightForHeaderInSection section: Int) -> CGFloat {
}
func tableView(_ tableView: UITableView, heightForFooterInSection section: Int) -> CGFloat {
}
func tableView(_ tableView: UITableView, heightForRowAt indexPath: IndexPath) -> CGFloat {
}
func tableView(_ tableView: UITableView, viewForHeaderInSection section: Int) -> UIView? {
}
func tableView(_ tableView: UITableView, viewForFooterInSection section: Int) -> UIView? {
}
func tableView(_ tableView: UITableView, didDeselectRowAt indexPath: IndexPath) {
}
func tableView(_ tableView: UITableView, editActionsForRowAt indexPath: IndexPath) -> [UITableViewRowAction]? {
}
func tableView(_ tableView: UITableView, canEditRowAt indexPath: IndexPath) -> Bool {
}
func tableView(_ tableView: UITableView, commit editingStyle: UITableViewCellEditingStyle, forRowAt indexPath: IndexPath) {
}
func tableView(_ tableView: UITableView, canMoveRowAt indexPath: IndexPath) -> Bool {
}
func tableView(_ tableView: UITableView, moveRowAt sourceIndexPath: IndexPath, to destinationIndexPath: IndexPath) {
}