UISearchController 搜尋控制器
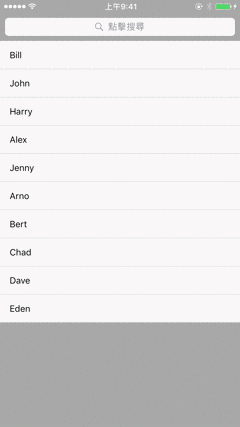
加入協定
class ViewControllertest: UIViewController, UITableViewDataSource, UITableViewDelegate, UISearchBarDelegate, UISearchResultsUpdating {
宣告資料
var search = UISearchController()
var tableview = UITableView()
var names = ["Bill", "John", "Harry", "Alex", "Jenny", "Arno", "Bert", "Chad", "Dave", "Eden"]
var searches: [String] = []
let WIDTH = UIScreen.main.bounds.size.width
let HEIGHT = UIScreen.main.bounds.size.height
viewDidLoad
view.backgroundColor = .lightGray
search = UISearchController(searchResultsController: nil)
search.searchBar.delegate = self
search.searchResultsUpdater = self
search.searchBar.searchBarStyle = .prominent
search.searchBar.placeholder = "點擊搜尋"
search.searchBar.setValue("取消",
forKey:"_cancelButtonText")
search.searchBar.tintColor = .white
search.searchBar.barTintColor = .lightGray
search.searchBar.backgroundImage = UIImage()
search.hidesNavigationBarDuringPresentation = true
search.dimsBackgroundDuringPresentation = false
search.searchBar.keyboardType = .default
search.searchBar.returnKeyType = .search
tableview = UITableView(frame: CGRect(x: 0,
y: 0,
width: WIDTH,
height: HEIGHT),
style: .plain)
tableview.delegate = self
tableview.dataSource = self
tableview.register(UITableViewCell.self,
forCellReuseIdentifier: "cell")
tableview.backgroundColor = .lightGray
tableview.separatorStyle = .singleLine
tableview.separatorColor = .lightGray
tableview.separatorInset = UIEdgeInsetsMake(0, 0, 0, 0)
tableview.tableHeaderView = search.searchBar
tableview.tableFooterView = UIView(frame: .zero)
view.addSubview(tableview)
UITableViewDelegate、UITableViewDatasource
func numberOfSections(in tableView: UITableView) -> Int {
return 1
}
func tableView(_ tableView: UITableView, numberOfRowsInSection section: Int) -> Int {
return search.isActive ? searches.count : names.count
}
func tableView(_ tableView: UITableView, cellForRowAt indexPath: IndexPath) -> UITableViewCell {
var cell = tableView.dequeueReusableCell(withIdentifier: "cell")
if cell == nil {
cell = UITableViewCell(style: .default,
reuseIdentifier: "cell")
}
cell?.textLabel?.text = search.isActive ? "\(searches[indexPath.row])" : "\(names[indexPath.row])"
return cell!
}
func tableView(_ tableView: UITableView, didSelectRowAt indexPath: IndexPath) {
tableView.deselectRow(at: indexPath,
animated: true)
}
建立更新搜尋動作
func updateSearchResults(for searchController: UISearchController) {
if let results = search.searchBar.text {
searches = names.filter { (name) -> Bool in
return name.contains(results)
}
}
tableview.reloadData()
}